このページでは、Chat アプリでダイアログを開いてユーザーに応答する方法について説明します。
ダイアログは、Chat スペースまたはメッセージから開く、カードベースのウィンドウ インターフェースです。ダイアログとその内容は、開いたユーザーにのみ表示されます。
チャットアプリは、ダイアログを使用して、マルチステップ フォームなど、Chat ユーザーから情報をリクエストして収集できます。フォーム入力の作成の詳細については、ユーザーからの情報の収集と処理をご覧ください。
前提条件
Node.js
インタラクティブ機能が有効になっている Google Chat アプリ。HTTP サービスを使用してインタラクティブな Chat アプリを作成するには、こちらのクイックスタートを完了してください。
Python
インタラクティブ機能が有効になっている Google Chat アプリ。HTTP サービスを使用してインタラクティブな Chat アプリを作成するには、こちらのクイックスタートを完了してください。
Java
インタラクティブ機能が有効になっている Google Chat アプリ。HTTP サービスを使用してインタラクティブな Chat アプリを作成するには、こちらのクイックスタートを完了してください。
Apps Script
インタラクティブ機能が有効になっている Google Chat アプリ。Apps Script でインタラクティブな Chat アプリを作成するには、こちらのクイックスタートを完了してください。
ダイアログを開く
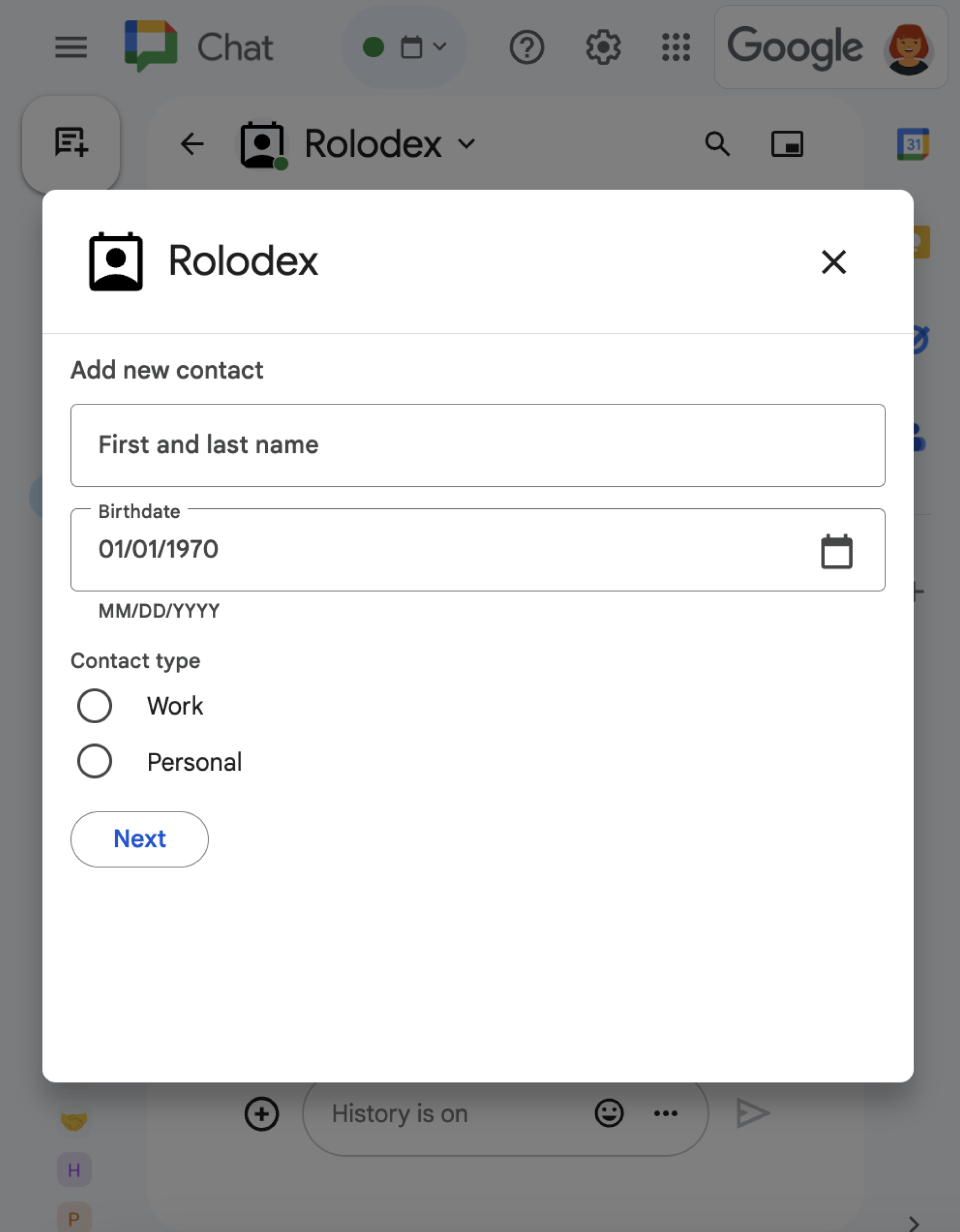
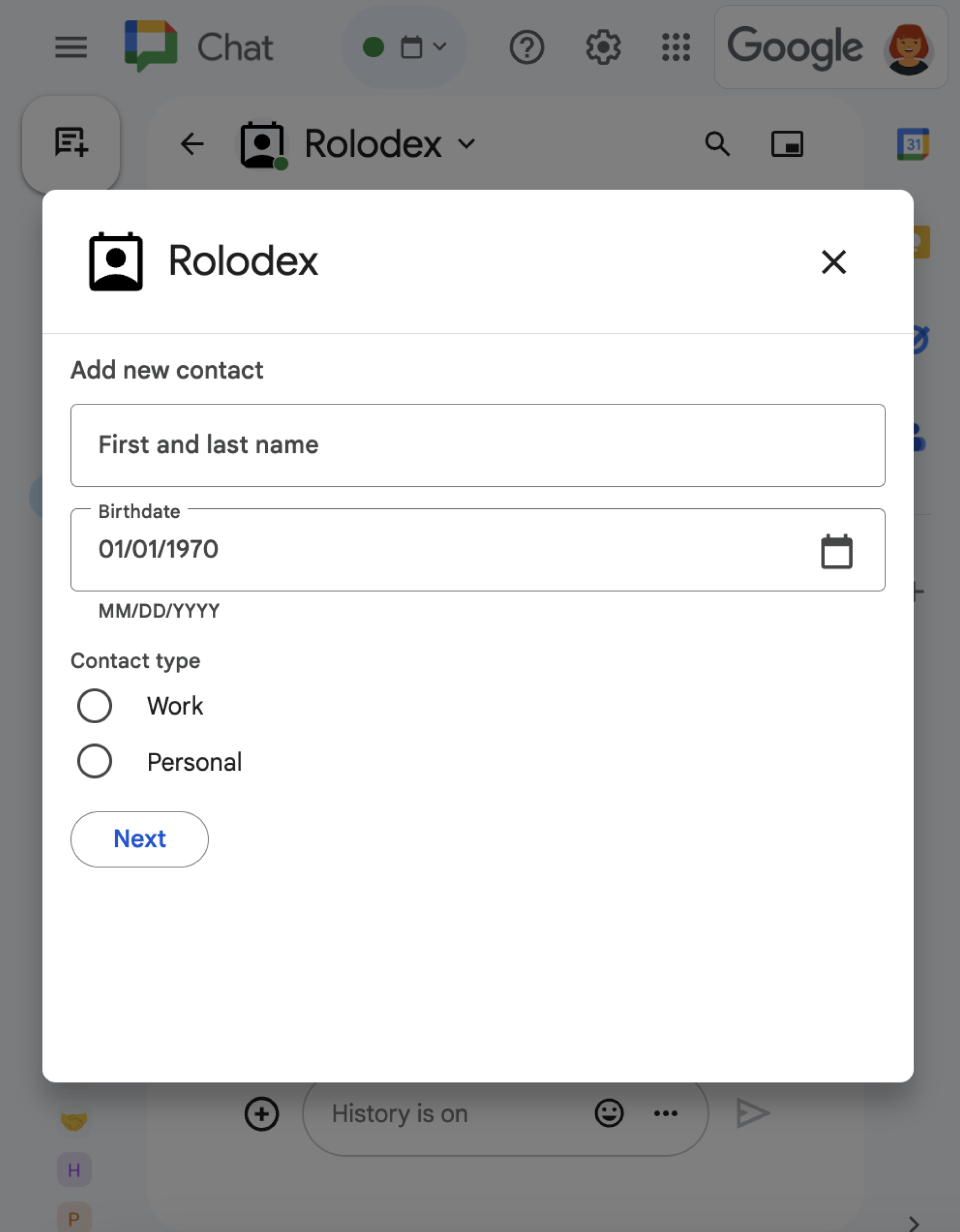
このセクションでは、次の手順で応答してダイアログを設定する方法について説明します。
- ユーザー操作からダイアログ リクエストをトリガーします。
- ダイアログを返して開き、リクエストを処理します。
- ユーザーが情報を送信したら、ダイアログを閉じるか、別のダイアログを返して送信を処理します。
ダイアログ リクエストをトリガーする
Chat アプリは、スラッシュ コマンドやカード内のメッセージからのボタンクリックなど、ユーザー操作に応答するためにダイアログを開くことができます。
ダイアログでユーザーに応答するには、Chat アプリでダイアログ リクエストをトリガーするインタラクションを作成する必要があります。次に例を示します。
- スラッシュ コマンドに返信する。スラッシュ コマンドからリクエストをトリガーするには、コマンドを構成するときに [Opens a dialog] チェックボックスをオンにする必要があります。
- メッセージ内のボタンクリックに応答します。カードの一部として、またはメッセージの下部に配置します。メッセージ内のボタンからリクエストをトリガーするには、ボタンの
interaction
をOPEN_DIALOG
に設定して、ボタンのonClick
アクションを構成します。 - Chat アプリのホームページでボタンのクリックに応答する。ホームページからダイアログを開く方法については、Google Chat アプリのホームページを作成するをご覧ください。
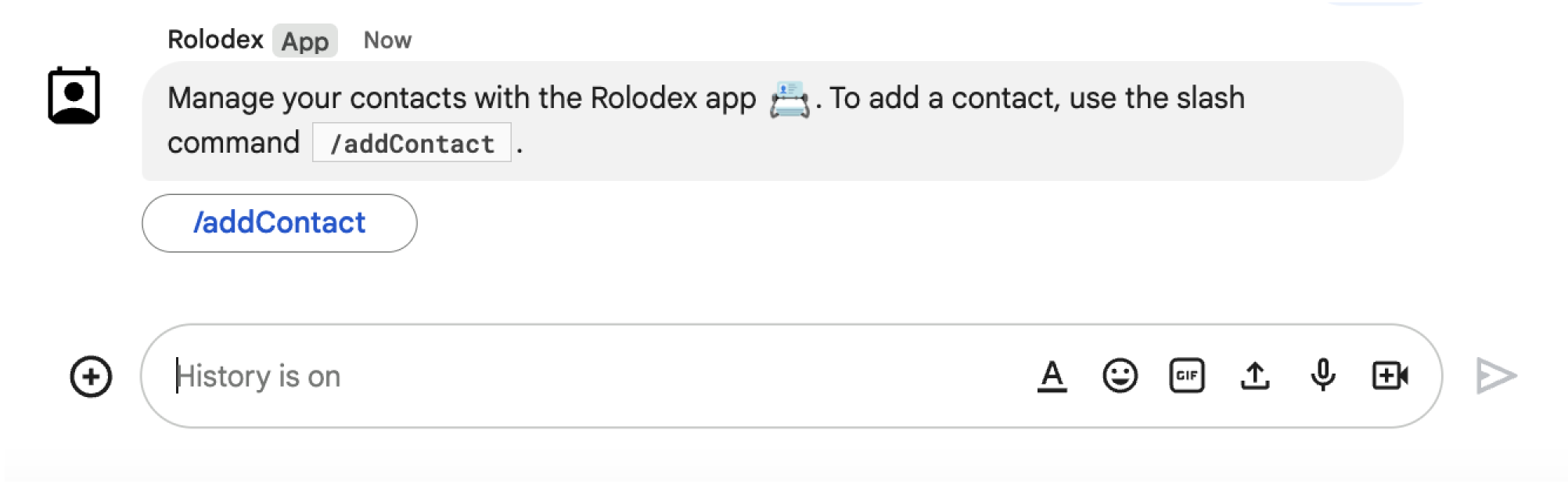
/addContact
スラッシュ コマンドの使用を求めるメッセージを送信しています。メッセージには、ユーザーがクリックしてコマンドをトリガーできるボタンも含まれています。
次のコードサンプルは、カード メッセージのボタンからダイアログ リクエストをトリガーする方法を示しています。ダイアログを開くには、button.interaction
フィールドを OPEN_DIALOG
に設定します。
Node.js
Python
Java
Apps Script
この例では、カード JSON を返すことでカード メッセージを送信します。Apps Script カード サービスを使用することもできます。
最初のダイアログを開く
ユーザーがダイアログ リクエストをトリガーすると、Chat アプリはインタラクション イベントを受信します。このイベントは、Chat API では event
型で表されます。インタラクションによってダイアログ リクエストがトリガーされると、イベントの dialogEventType
フィールドは REQUEST_DIALOG
に設定されます。
ダイアログを開くには、type
を DIALOG
に設定した actionResponse
オブジェクトと Message
オブジェクトを返すことで、Chat アプリがリクエストに応答します。ダイアログの内容を指定するには、次のオブジェクトを含めます。
type
がDIALOG
に設定されたactionResponse
オブジェクト。dialogAction
オブジェクト。body
フィールドには、カードに表示するユーザー インターフェース(UI)要素(1 つ以上のsections
ウィジェットなど)が含まれます。ユーザーから情報を収集するには、フォーム入力ウィジェットとボタン ウィジェットを指定します。フォーム入力の設計の詳細については、ユーザーから情報を収集して処理するをご覧ください。
次のコードサンプルは、Chat アプリがダイアログを開くレスポンスを返す方法を示しています。
Node.js
Python
Java
Apps Script
この例では、カード JSON を返すことでカード メッセージを送信します。Apps Script カード サービスを使用することもできます。
ダイアログの送信を処理する
ユーザーがダイアログを送信するボタンをクリックすると、Chat アプリは CARD_CLICKED
インタラクション イベントを受け取ります。ここで、dialogEventType
は SUBMIT_DIALOG
です。
Chat アプリは、次のいずれかの方法でインタラクション イベントを処理する必要があります。
- 別のダイアログを返す: 別のカードまたはフォームに入力します。
- ユーザーが送信したデータを検証したら、ダイアログを閉じ、必要に応じて確認メッセージを送信します。
省略可: 別のダイアログを返す
ユーザーが最初のダイアログを送信すると、Chat アプリは 1 つ以上の追加ダイアログを返すことができます。これにより、ユーザーは送信前に情報を確認したり、複数のステップからなるフォームを完了したり、フォームのコンテンツを動的に入力したりできます。
ユーザーが入力したデータを処理するために、Chat アプリは event.common.formInputs
オブジェクトを使用します。入力ウィジェットから値を取得する方法については、ユーザーから情報を収集して処理するをご覧ください。
ユーザーが最初のダイアログから入力したデータを追跡するには、次のダイアログを開くボタンにパラメータを追加する必要があります。詳しくは、別のカードにデータを移行するをご覧ください。
この例では、Chat アプリが最初のダイアログを開き、送信前に確認を求める 2 番目のダイアログが表示されます。
Node.js
Python
Java
Apps Script
この例では、カード JSON を返すことでカード メッセージを送信します。Apps Script カード サービスを使用することもできます。
ダイアログを閉じる
ユーザーがダイアログのボタンをクリックすると、Chat アプリは関連するアクションを実行し、イベント オブジェクトに次の情報を提供します。
eventType
はCARD_CLICKED
です。dialogEventType
はSUBMIT_DIALOG
です。
Chat アプリは、type
が DIALOG
と dialogAction
に設定された ActionResponse
オブジェクトを返す必要があります。
省略可: 通知を表示する
ダイアログを閉じるときにテキスト通知を表示することもできます。
Chat アプリは、actionStatus
が設定された ActionResponse
を返すことで、成功またはエラーの通知を返すことができます。
次の例では、パラメータが有効であることを確認して、結果に応じてテキスト通知でダイアログを閉じます。
Node.js
Python
Java
Apps Script
この例では、カード JSON を返すことでカード メッセージを送信します。Apps Script カード サービスを使用することもできます。
ダイアログ間でパラメータを渡す方法については、別のカードにデータを転送するをご覧ください。
省略可: 確認メッセージを送信する
ダイアログを閉じるときに、新しいメッセージを送信したり、既存のメッセージを更新したりすることもできます。
新しいメッセージを送信するには、type
を NEW_MESSAGE
に設定した ActionResponse
オブジェクトを返します。次の例では、テキスト通知と確認テキスト メッセージでダイアログを閉じます。
Node.js
Python
Java
Apps Script
この例では、カード JSON を返すことでカード メッセージを送信します。Apps Script カード サービスを使用することもできます。
メッセージを更新するには、更新されたメッセージを含む actionResponse
オブジェクトを返します。type
は次のいずれかに設定します。
UPDATE_MESSAGE
: ダイアログ リクエストをトリガーしたメッセージを更新します。UPDATE_USER_MESSAGE_CARDS
: リンク プレビューからカードを更新します。
トラブルシューティング
Google Chat アプリまたはカードからエラーが返されると、Chat インターフェースに「エラーが発生しました」というメッセージが表示されます。または「リクエストを処理できません」というメッセージが表示されます。Chat UI にエラー メッセージが表示されない場合でも、Chat アプリまたはカードで予期しない結果が生成されることがあります(カード メッセージが表示されないなど)。
チャット UI にエラー メッセージが表示されない場合でも、チャットアプリのエラー ロギングがオンになっている場合は、エラーの修正に役立つ説明的なエラー メッセージとログデータが利用できます。エラーの表示、デバッグ、修正については、Google Chat エラーのトラブルシューティングと修正をご覧ください。
関連トピック
- 連絡先マネージャーのサンプルを確認する。これは、ダイアログを使用して連絡先情報を収集する Chat アプリです。
- Google Chat アプリのホームページからダイアログを開く。
- スラッシュ コマンドを設定して応答する
- ユーザーが入力した情報を処理する