Esta página descreve como o app de chat pode abrir caixas de diálogo para responder aos usuários.
As caixas de diálogo são interfaces com base em cards que são abertas em um espaço ou mensagem do Chat. A caixa de diálogo e o conteúdo dela só ficam visíveis para o usuário que a abriu.
Os apps de chat podem usar caixas de diálogo para solicitar e coletar informações dos usuários, incluindo formulários de várias etapas. Para mais detalhes sobre como criar entradas de formulário, consulte Coletar e processar informações dos usuários.
Pré-requisitos
Node.js
Um app do Google Chat com recursos interativos ativados. Para criar um app de chat interativo usando um serviço HTTP, conclua este guia de início rápido.
Python
Um app do Google Chat com recursos interativos ativados. Para criar um app de chat interativo usando um serviço HTTP, conclua este guia de início rápido.
Java
Um app do Google Chat com recursos interativos ativados. Para criar um app de chat interativo usando um serviço HTTP, conclua este guia de início rápido.
Apps Script
Um app do Google Chat com recursos interativos ativados. Para criar um app do Chat interativo no Apps Script, conclua este guia de início rápido.
Abrir uma caixa de diálogo
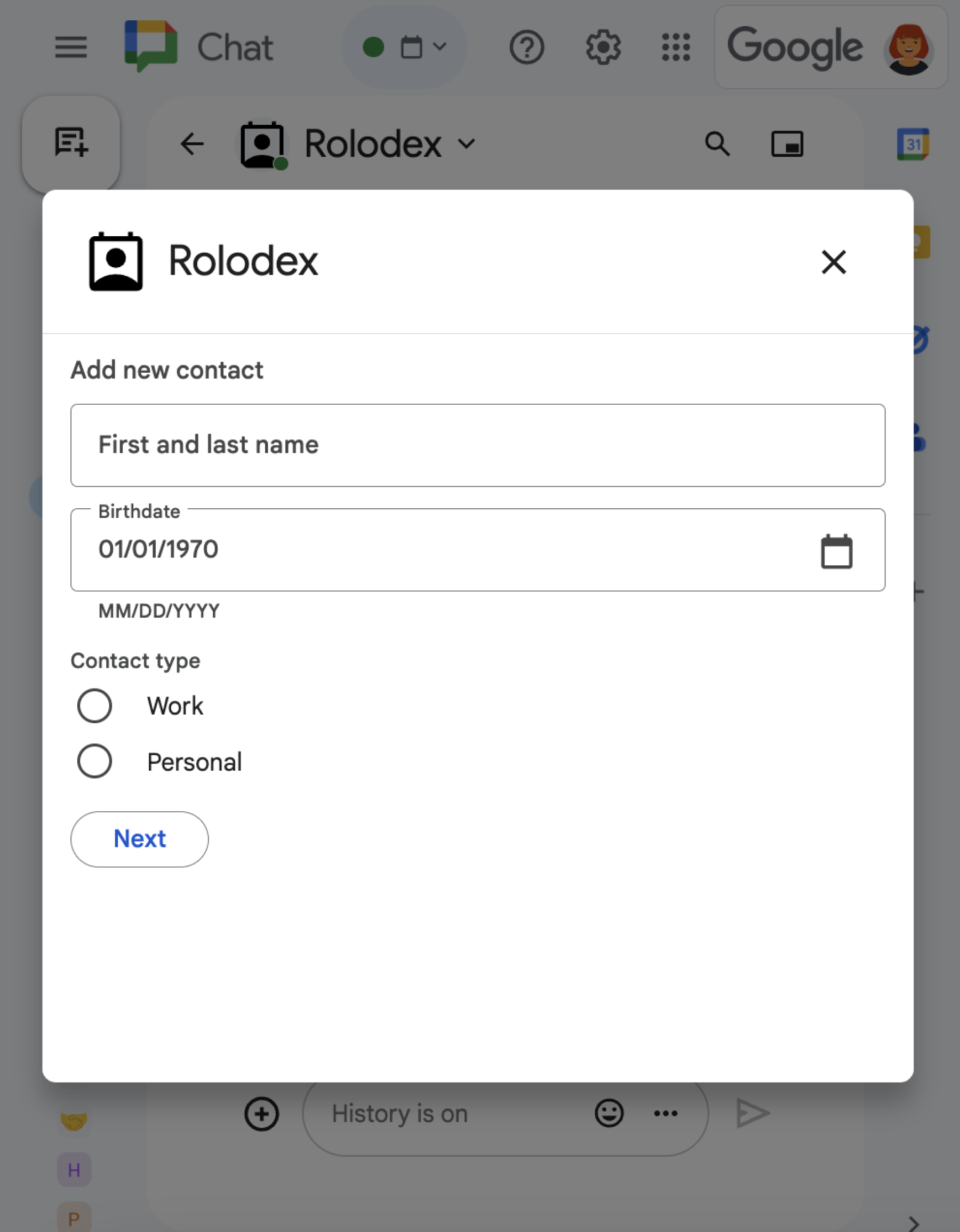
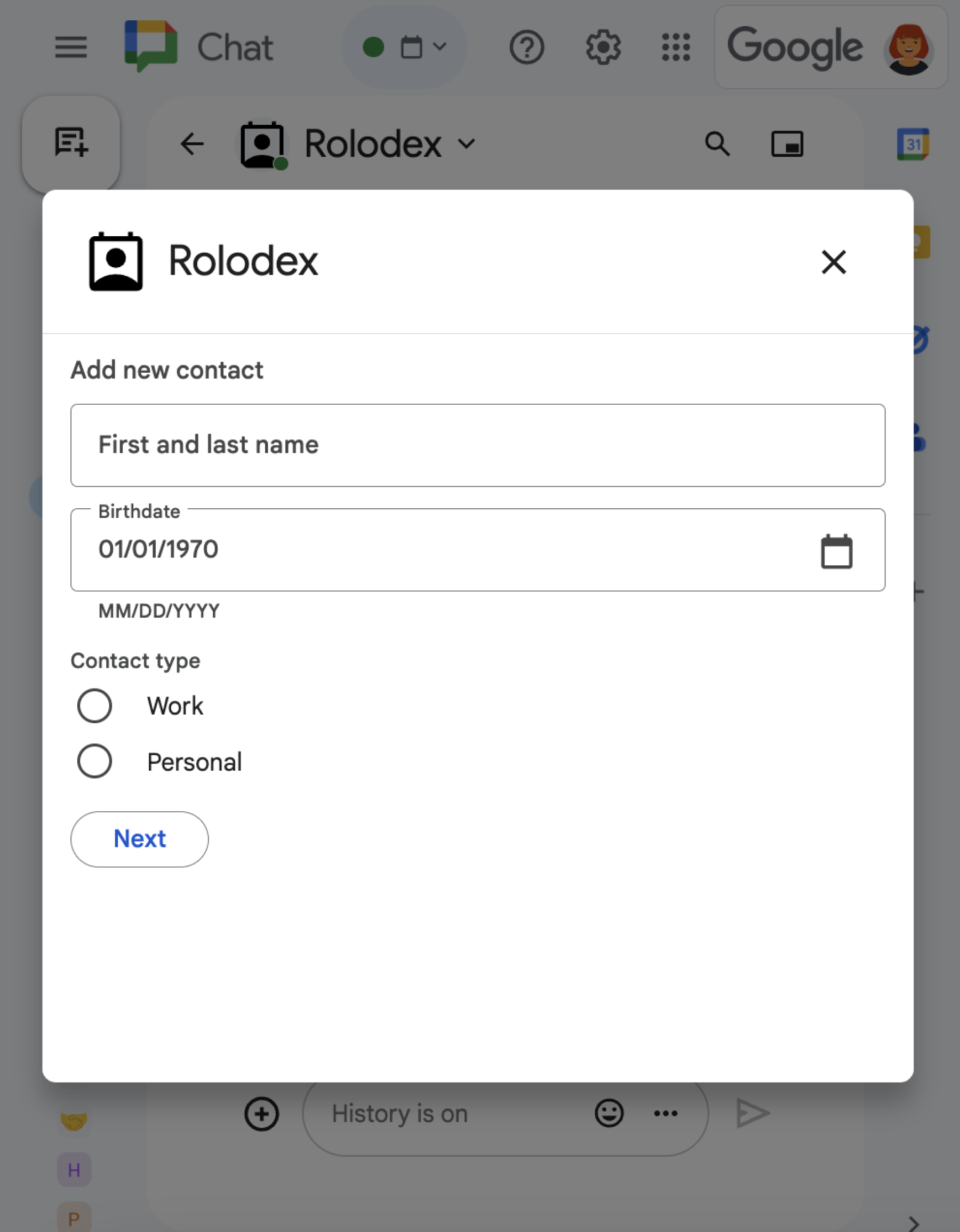
Esta seção explica como responder e configurar uma caixa de diálogo:
- Acionar a solicitação de caixa de diálogo de uma interação do usuário.
- Processe a solicitação retornando e abrindo uma caixa de diálogo.
- Depois que os usuários enviarem informações, processe o envio fechando a caixa de diálogo ou retornando outra caixa de diálogo.
Acionar uma solicitação de caixa de diálogo
Um app de chat só pode abrir caixas de diálogo para responder a uma interação do usuário, como um comando de barra ou um clique em um botão de uma mensagem em um card.
Para responder aos usuários com uma caixa de diálogo, um app de chat precisa criar uma interação que aciona a solicitação de caixa de diálogo, como esta:
- Responder a um comando de barra. Para acionar a solicitação de um comando de barra, marque a caixa de seleção Abre uma caixa de diálogo ao configurar o comando.
- Responda a um clique de botão em uma
mensagem,
seja como parte de um card ou na parte de baixo da mensagem. Para acionar a
solicitação de um botão em uma mensagem, configure a
ação
onClick
do botão definindo ointeraction
comoOPEN_DIALOG
. - Responder a um clique de botão na página inicial de um app de chat. Para saber como abrir caixas de diálogo nas páginas iniciais, consulte Criar uma página inicial para o app Google Chat.
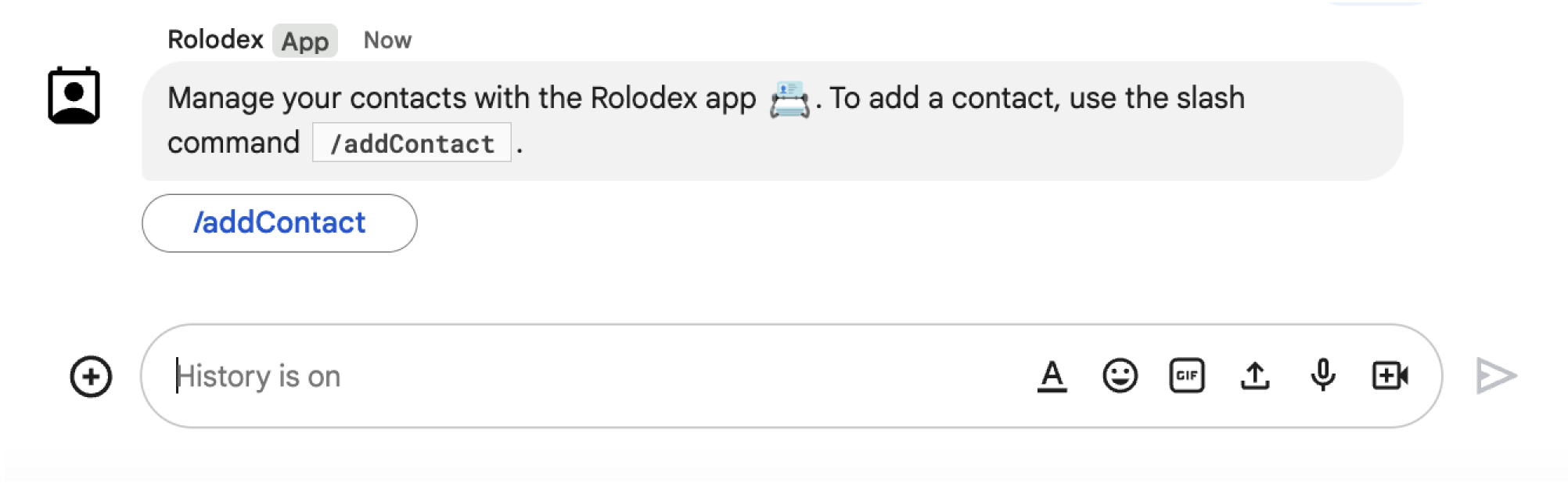
/addContact
. A mensagem também inclui um botão que os usuários podem clicar para acionar o comando.
O exemplo de código a seguir mostra como acionar uma solicitação de caixa de diálogo de um botão em
uma mensagem de cartão. Para abrir a caixa de diálogo, o campo
button.interaction
é definido como OPEN_DIALOG
:
Node.js
Python
Java
Apps Script
Este exemplo envia uma mensagem de cartão retornando o JSON do cartão. Também é possível usar o serviço de cards do Apps Script.
Abrir a caixa de diálogo inicial
Quando um usuário aciona uma solicitação de caixa de diálogo, o app Chat
recebe um evento de interação, representado como um tipo
event
na
API Chat. Se a interação acionar uma solicitação de caixa de diálogo, o campo
dialogEventType
do evento será definido como REQUEST_DIALOG
.
Para abrir uma caixa de diálogo, o app de chat pode responder à
solicitação retornando um objeto
actionResponse
com o type
definido como DIALOG
e o
objeto
Message
. Para especificar o conteúdo da caixa de diálogo, inclua os seguintes
objetos:
- Um objeto
actionResponse
comtype
definido comoDIALOG
. - Um objeto
dialogAction
. O campobody
contém os elementos da interface do usuário (IU) a serem exibidos no card, incluindo um ou maissections
de widgets. Para coletar informações dos usuários, você pode especificar widgets de entrada de formulário e um widget de botão. Para saber mais sobre como projetar entradas de formulário, consulte Coletar e processar informações dos usuários.
O exemplo de código abaixo mostra como um app de chat retorna uma resposta que abre uma caixa de diálogo:
Node.js
Python
Java
Apps Script
Este exemplo envia uma mensagem de cartão retornando o JSON do cartão. Também é possível usar o serviço de cards do Apps Script.
Processar o envio da caixa de diálogo
Quando os usuários clicam em um botão que envia uma caixa de diálogo, o
app de chat recebe
um evento de interação CARD_CLICKED
,
em que dialogEventType
é SUBMIT_DIALOG
.
O app de chat precisa processar o evento de interação fazendo uma das seguintes ações:
- Retorne outra caixa de diálogo para preencher outro card ou formulário.
- Feche a caixa de diálogo depois de validar os dados enviados pelo usuário e, se quiser, envie uma mensagem de confirmação.
Opcional: retornar outra caixa de diálogo
Depois que os usuários enviam a caixa de diálogo inicial, os apps de chat podem retornar uma ou mais caixas de diálogo adicionais para ajudar os usuários a revisar informações antes de enviar, preencher formulários de várias etapas ou preencher o conteúdo do formulário de forma dinâmica.
Para processar os dados inseridos pelos usuários, o app Chat
usa o
objeto
event.common.formInputs
. Para saber mais sobre como recuperar valores de widgets de entrada, consulte
Coletar e processar informações dos usuários.
Para acompanhar os dados que os usuários inserem na caixa de diálogo inicial, adicione parâmetros ao botão que abre a próxima caixa de diálogo. Para mais detalhes, consulte Transferir dados para outro cartão.
Neste exemplo, um app de chat abre uma caixa de diálogo inicial que leva a uma segunda caixa de diálogo para confirmação antes do envio:
Node.js
Python
Java
Apps Script
Este exemplo envia uma mensagem de cartão retornando o JSON do cartão. Também é possível usar o serviço de cards do Apps Script.
Fechar a caixa de diálogo
Quando os usuários clicam em um botão em uma caixa de diálogo, o app de chat executa a ação associada e fornece ao objeto de evento as seguintes informações:
eventType
éCARD_CLICKED
.dialogEventType
éSUBMIT_DIALOG
.
O app de chat precisa retornar um objeto
ActionResponse
com o type
definido como DIALOG
e dialogAction
.
Opcional: mostrar uma notificação
Ao fechar a caixa de diálogo, você também pode mostrar uma notificação de texto.
O app de chat pode responder com uma notificação de sucesso ou erro
retornando um
ActionResponse
com actionStatus
definido.
O exemplo a seguir verifica se os parâmetros são válidos e fecha a caixa de diálogo com uma notificação de texto, dependendo do resultado:
Node.js
Python
Java
Apps Script
Este exemplo envia uma mensagem de cartão retornando o JSON do cartão. Também é possível usar o serviço de cards do Apps Script.
Para mais detalhes sobre a transmissão de parâmetros entre caixas de diálogo, consulte Transferir dados para outro card.
Opcional: enviar uma mensagem de confirmação
Ao fechar a caixa de diálogo, você também pode enviar uma nova mensagem ou atualizar uma existente.
Para enviar uma nova mensagem, retorne um objeto
ActionResponse
com o type
definido como NEW_MESSAGE
. O exemplo a seguir fecha a
caixa de diálogo com a notificação de texto e a mensagem de texto de confirmação:
Node.js
Python
Java
Apps Script
Este exemplo envia uma mensagem de cartão retornando o JSON do cartão. Também é possível usar o serviço de cards do Apps Script.
Para atualizar uma mensagem, retorne um objeto actionResponse
que contenha a
mensagem atualizada e defina o type
como uma das seguintes opções:
UPDATE_MESSAGE
: atualiza a mensagem que acionou a solicitação de caixa de diálogo.UPDATE_USER_MESSAGE_CARDS
: atualiza o card de uma prévia de link.
Resolver problemas
Quando um app do Google Chat ou um card retorna um erro, a interface do Chat mostra uma mensagem informando que "Ocorreu um erro". ou "Não foi possível processar sua solicitação". Às vezes, a interface do Chat não mostra nenhuma mensagem de erro, mas o app ou o card do Chat produz um resultado inesperado. Por exemplo, uma mensagem de card pode não aparecer.
Embora uma mensagem de erro possa não aparecer na interface do Chat, mensagens de erro descritivas e dados de registro estão disponíveis para ajudar a corrigir erros quando o registro de erros para apps de chat estiver ativado. Para saber como visualizar, depurar e corrigir erros, consulte Resolver e corrigir erros do Google Chat.
Temas relacionados
- Confira o exemplo do Gerenciador de contatos, um app de chat que usa caixas de diálogo para coletar informações de contato.
- Abrir caixas de diálogo na página inicial do app Google Chat.
- Configurar e responder a comandos de barra
- Processar informações inseridas pelos usuários