Panduan ini menjelaskan cara memanggil API Google Chat
messages.create()
metode untuk melakukan salah satu tindakan berikut:
- Kirim pesan yang berisi teks, kartu, dan widget interaktif.
- Mengirim pesan secara pribadi kepada pengguna Chat tertentu.
- Memulai atau membalas rangkaian pesan.
- Beri nama pesan sehingga Anda dapat menentukannya di Chat API lain permintaan.
Ukuran pesan maksimum (termasuk teks atau kartu apa pun) adalah 32.000 byte. Untuk mengirim pesan yang melebihi ukuran ini, aplikasi Chat Anda harus mengirim beberapa pesan.
Selain memanggil metode messages.create()
, aplikasi Chat
dapat membuat dan mengirim pesan untuk membalas interaksi pengguna, seperti memposting
pesan selamat datang setelah pengguna menambahkan aplikasi Chat ke
spasi. Saat merespons interaksi, aplikasi Chat dapat menggunakan
jenis fitur pesan, termasuk dialog interaktif dan pratinjau link
antarmuka. Untuk membalas pengguna, aplikasi Chat akan kembali
pesan secara sinkron, tanpa memanggil Chat API. Untuk mempelajari
tentang mengirim pesan untuk merespons interaksi, lihat
Menerima dan merespons interaksi dengan aplikasi Google Chat.
Cara Chat menampilkan dan mengatribusikan pesan yang dibuat dengan Chat API
Anda dapat memanggil metode messages.create()
menggunakan
autentikasi aplikasi
dan autentikasi pengguna.
Chat mengatribusikan pengirim pesan secara berbeda
tergantung pada jenis
otentikasi yang Anda gunakan.
Saat Anda melakukan autentikasi sebagai aplikasi Chat, aplikasi Chat mengirimkan pesan.
App
di samping namanya.Saat Anda melakukan autentikasi sebagai pengguna, aplikasi Chat akan mengirim pesan atas nama pengguna. Chat juga mengaitkan Aplikasi Chat ke pesan dengan menampilkan namanya.
Jenis otentikasi juga menentukan fitur dan antarmuka pesan mana yang dapat Anda sertakan dalam pesan tersebut. Dengan otentikasi aplikasi, Aplikasi chat dapat mengirim pesan yang berisi teks kaya, antarmuka berbasis kartu, dan {i>widget<i} interaktif. Karena pengguna Chat hanya dapat mengirim teks dalam pesan mereka, Anda dapat hanya menyertakan teks saat membuat pesan menggunakan otentikasi pengguna. Untuk mempelajari fitur pesan lebih lanjut yang tersedia untuk Chat API, lihat Ringkasan pesan Google Chat.
Panduan ini menjelaskan cara menggunakan salah satu jenis autentikasi untuk mengirim pesan dengan Chat API.
Prasyarat
Node.js
- Business atau Enterprise Akun Google Workspace yang memiliki akses ke Google Chat.
- Menyiapkan lingkungan Anda:
- Buat project Google Cloud.
- Konfigurasi layar izin OAuth.
- Aktifkan dan konfigurasikan Google Chat API dengan nama, ikon, dan deskripsi untuk aplikasi Chat Anda.
- Instal Node.js Library Klien Cloud.
- Buat kredensial akses berdasarkan cara Anda ingin melakukan autentikasi di Google Chat API
permintaan:
- Untuk melakukan autentikasi sebagai pengguna Chat,
buat client ID OAuth
kredensial, lalu simpan kredensial sebagai file JSON yang bernama
client_secrets.json
ke direktori lokal Anda. - Untuk melakukan autentikasi sebagai aplikasi Chat,
buat akun layanan
kredensial, lalu simpan kredensial sebagai file JSON yang bernama
credentials.json
.
- Untuk melakukan autentikasi sebagai pengguna Chat,
buat client ID OAuth
kredensial, lalu simpan kredensial sebagai file JSON yang bernama
- Pilih cakupan otorisasi berdasarkan apakah Anda ingin melakukan autentikasi sebagai pengguna atau Aplikasi Chat.
- Ruang Google Chat tempat pengguna yang diautentikasi atau memanggil aplikasi Chat adalah anggotanya. Untuk mengotentikasi sebagai aplikasi Chat, tambahkan Chat ke ruang.
Python
- Business atau Enterprise Akun Google Workspace yang memiliki akses ke Google Chat.
- Menyiapkan lingkungan Anda:
- Buat project Google Cloud.
- Konfigurasi layar izin OAuth.
- Aktifkan dan konfigurasikan Google Chat API dengan nama, ikon, dan deskripsi untuk aplikasi Chat Anda.
- Instal Python Library Klien Cloud.
- Buat kredensial akses berdasarkan cara Anda ingin melakukan autentikasi di Google Chat API
permintaan:
- Untuk melakukan autentikasi sebagai pengguna Chat,
buat client ID OAuth
kredensial, lalu simpan kredensial sebagai file JSON yang bernama
client_secrets.json
ke direktori lokal Anda. - Untuk melakukan autentikasi sebagai aplikasi Chat,
buat akun layanan
kredensial, lalu simpan kredensial sebagai file JSON yang bernama
credentials.json
.
- Untuk melakukan autentikasi sebagai pengguna Chat,
buat client ID OAuth
kredensial, lalu simpan kredensial sebagai file JSON yang bernama
- Pilih cakupan otorisasi berdasarkan apakah Anda ingin melakukan autentikasi sebagai pengguna atau Aplikasi Chat.
- Ruang Google Chat tempat pengguna yang diautentikasi atau memanggil aplikasi Chat adalah anggotanya. Untuk mengotentikasi sebagai aplikasi Chat, tambahkan Chat ke ruang.
Java
- Business atau Enterprise Akun Google Workspace yang memiliki akses ke Google Chat.
- Menyiapkan lingkungan Anda:
- Buat project Google Cloud.
- Konfigurasi layar izin OAuth.
- Aktifkan dan konfigurasikan Google Chat API dengan nama, ikon, dan deskripsi untuk aplikasi Chat Anda.
- Instal Java Library Klien Cloud.
- Buat kredensial akses berdasarkan cara Anda ingin melakukan autentikasi di Google Chat API
permintaan:
- Untuk melakukan autentikasi sebagai pengguna Chat,
buat client ID OAuth
kredensial, lalu simpan kredensial sebagai file JSON yang bernama
client_secrets.json
ke direktori lokal Anda. - Untuk melakukan autentikasi sebagai aplikasi Chat,
buat akun layanan
kredensial, lalu simpan kredensial sebagai file JSON yang bernama
credentials.json
.
- Untuk melakukan autentikasi sebagai pengguna Chat,
buat client ID OAuth
kredensial, lalu simpan kredensial sebagai file JSON yang bernama
- Pilih cakupan otorisasi berdasarkan apakah Anda ingin melakukan autentikasi sebagai pengguna atau Aplikasi Chat.
- Ruang Google Chat tempat pengguna yang diautentikasi atau memanggil aplikasi Chat adalah anggotanya. Untuk mengotentikasi sebagai aplikasi Chat, tambahkan Chat ke ruang.
Apps Script
- Business atau Enterprise Akun Google Workspace yang memiliki akses ke Google Chat.
- Menyiapkan lingkungan Anda:
- Buat project Google Cloud.
- Konfigurasi layar izin OAuth.
- Aktifkan dan konfigurasikan Google Chat API dengan nama, ikon, dan deskripsi untuk aplikasi Chat Anda.
- Membuat project Apps Script mandiri, dan aktifkan Layanan Chat Lanjutan.
- Dalam panduan ini, Anda harus menggunakan pengguna atau autentikasi aplikasi. Untuk melakukan autentikasi sebagai aplikasi Chat, buat dan kredensial akun layanan. Untuk mengetahui langkah-langkahnya, lihat Autentikasi dan otorisasi sebagai Aplikasi Google Chat.
- Pilih cakupan otorisasi berdasarkan apakah Anda ingin melakukan autentikasi sebagai pengguna atau Aplikasi Chat.
- Ruang Google Chat tempat pengguna yang diautentikasi atau memanggil aplikasi Chat adalah anggotanya. Untuk mengotentikasi sebagai aplikasi Chat, tambahkan Chat ke ruang.
Mengirim pesan sebagai aplikasi Chat
Bagian ini menjelaskan cara mengirim pesan yang berisi teks, kartu, dan widget aksesori interaktif menggunakan autentikasi aplikasi.
Untuk memanggil messages.create()
menggunakan autentikasi aplikasi, Anda harus menentukan
kolom berikut dalam permintaan:
- Cakupan otorisasi
chat.bot
. - Resource
Space
tempat tempat Anda ingin memposting pesan. Aplikasi Chat harus anggota ruang. Message
resource yang akan dibuat. Untuk menentukan isi pesan, Anda dapat menyertakan teks kaya (text
), satu atau beberapa antarmuka kartu (cardsV2
), atau keduanya.
Secara opsional, Anda dapat menyertakan hal berikut:
- Kolom
accessoryWidgets
yang akan disertakan tombol interaktif di bagian bawah pesan. - Kolom
privateMessageViewer
untuk mengirim pesan secara pribadi ke pengguna tertentu. - Kolom
messageId
, yang memungkinkan Anda memberi nama pesan yang akan digunakan dalam permintaan API lainnya. - Kolom
thread.threadKey
danmessageReplyOption
untuk memulai atau membalas rangkaian pesan. Jika ruang tidak menggunakan rangkaian pesan, kolom ini akan diabaikan.
Kode berikut menunjukkan contoh cara aplikasi Chat dapat mengirim pesan yang diposting sebagai aplikasi Chat yang berisi teks, kartu, dan tombol yang dapat diklik di bagian bawah pesan:
Node.js
Python
Java
Apps Script
Untuk menjalankan contoh ini, ganti SPACE_NAME
dengan ID dari
ruang
Kolom name
.
Anda bisa mendapatkan ID dengan memanggil
Metode spaces.list()
atau dari URL ruang.
Menambahkan widget interaktif di bagian bawah pesan
Dalam contoh kode pertama dari panduan ini, Pesan aplikasi Chat menampilkan tombol yang dapat diklik di bagian bawah pesan, yang dikenal sebagai widget aksesori. Widget aksesori akan muncul setelah teks atau kartu dalam pesan. Anda dapat menggunakan widget ini untuk berinteraksi dengan pesan Anda dalam banyak cara, termasuk hal berikut:
- Memberi rating akurasi atau kepuasan pesan.
- Laporkan masalah terkait pesan atau aplikasi Chat.
- Buka link ke konten terkait, seperti dokumentasi.
- Menutup atau menunda pesan serupa dari aplikasi Chat selama jangka waktu tertentu.
Untuk menambahkan widget aksesori, sertakan
accessoryWidgets[]
di isi permintaan Anda dan tentukan satu atau beberapa widget yang diinginkan
untuk disertakan.
Gambar berikut menunjukkan aplikasi Chat yang menambahkan pesan teks dengan widget aksesori agar pengguna dapat menilai pengalaman mereka dengan aplikasi Chat.
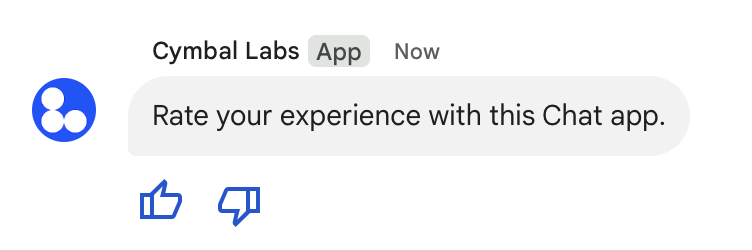
Berikut ini adalah isi permintaan yang membuat pesan teks dengan
dua tombol aksesori. Saat pengguna mengeklik tombol, atribut
(seperti doUpvote
) memproses interaksi:
{
text: "Rate your experience with this Chat app.",
accessoryWidgets: [{ buttonList: { buttons: [{
icon: { material_icon: {
name: "thumb_up"
}},
color: { red: 0, blue: 255, green: 0 },
onClick: { action: {
function: "doUpvote"
}}
}, {
icon: { material_icon: {
name: "thumb_down"
}},
color: { red: 0, blue: 255, green: 0 },
onClick: { action: {
function: "doDownvote"
}}
}]}}]
}
Mengirim pesan secara pribadi
Aplikasi Chat dapat mengirim pesan secara pribadi sehingga pesan hanya dapat dilihat oleh pengguna tertentu dalam ruang. Ketika seorang Aplikasi Chat mengirimkan pesan pribadi, yaitu pesan menampilkan label yang memberi tahu pengguna bahwa pesan tersebut hanya dapat dilihat oleh mereka.
Untuk mengirim pesan secara pribadi menggunakan Chat API, tentukan atribut
privateMessageViewer
di isi permintaan Anda. Untuk menentukan pengguna, Anda menetapkan nilai ke
resource User
yang
mewakili pengguna Chat. Anda juga dapat menggunakan
Kolom name
halaman
User
, seperti yang ditunjukkan dalam contoh berikut:
{
text: "Hello private world!",
privateMessageViewer: {
name: "users/USER_ID"
}
}
Untuk menggunakan contoh ini, ganti USER_ID
dengan ID unik untuk pengguna tersebut, seperti 12345678987654321
atau
hao@cymbalgroup.com
. Untuk informasi selengkapnya tentang menentukan pengguna, lihat
Mengidentifikasi dan menentukan pengguna Google Chat.
Untuk mengirim pesan secara pribadi, Anda harus menghapus hal berikut dalam permintaan:
Mengirim pesan teks atas nama pengguna
Bagian ini menjelaskan cara mengirim pesan atas nama pengguna menggunakan autentikasi pengguna. Dengan autentikasi pengguna, isi pesan hanya dapat berisi teks dan wajib menghapus fitur pesan yang hanya tersedia untuk Aplikasi chat, termasuk antarmuka kartu dan widget interaktif.
Untuk memanggil messages.create()
menggunakan autentikasi pengguna, Anda harus menentukan
kolom berikut dalam permintaan:
- Cakupan otorisasi
yang mendukung otentikasi
pengguna untuk metode ini. Contoh berikut menggunakan
cakupan
chat.messages.create
. - Resource
Space
tempat tempat Anda ingin memposting pesan. Pengguna terautentikasi haruslah anggota spasi. Message
resource yang akan dibuat. Untuk mendefinisikan isi pesan, Anda harus menyertakan atributtext
kolom tersebut.
Secara opsional, Anda dapat menyertakan hal berikut:
- Kolom
messageId
, yang memungkinkan Anda memberi nama pesan yang akan digunakan dalam permintaan API lainnya. - Kolom
thread.threadKey
danmessageReplyOption
untuk memulai atau membalas rangkaian pesan. Jika ruang tidak menggunakan rangkaian pesan, kolom ini akan diabaikan.
Kode berikut menunjukkan contoh cara aplikasi Chat dapat mengirim pesan teks di ruang tertentu atas nama pengguna terautentikasi:
Node.js
Python
Java
Apps Script
Untuk menjalankan contoh ini, ganti SPACE_NAME
dengan ID dari
ruang
name
kolom tersebut. Anda bisa mendapatkan ID dengan memanggil
Metode spaces.list()
atau dari URL ruang.
Memulai atau membalas dalam rangkaian pesan
Untuk ruang yang menggunakan thread, Anda dapat menentukan apakah pesan baru memulai rangkaian pesan, atau membalas ke rangkaian pesan yang sudah ada.
Secara default, pesan yang Anda buat menggunakan Chat API akan memulai . Untuk membantu Anda mengidentifikasi rangkaian pesan dan membalasnya nanti, Anda dapat menentukan kunci thread dalam permintaan Anda:
- Dalam isi permintaan Anda, tentukan
thread.threadKey
kolom tersebut. - Menentukan parameter kueri
messageReplyOption
untuk menentukan apa yang akan terjadi jika kunci tersebut sudah ada.
Untuk membuat pesan yang membalas rangkaian pesan yang ada:
- Dalam isi permintaan Anda, sertakan kolom
thread
. Jika diatur, Anda dapat menentukanthreadKey
yang Anda buat. Jika tidak, Anda harus menggunakanname
dari utas. - Tentukan parameter kueri
messageReplyOption
.
Kode berikut menunjukkan contoh cara aplikasi Chat dapat mengirim pesan teks yang dimulai atau membalas ke utas tertentu yang diidentifikasi oleh kunci dari ruang tertentu atas nama pengguna terautentikasi:
Node.js
Python
Java
Apps Script
Untuk menjalankan contoh ini, ganti kode berikut:
THREAD_KEY
: kunci thread yang ada dalam ruang, atau untuk membuat thread baru, nama unik untuk thread.SPACE_NAME
: ID dariname
kolom tersebut. Anda bisa mendapatkan ID dengan memanggil Metodespaces.list()
atau dari URL ruang.
Memberi nama pesan
Untuk mengambil atau menetapkan pesan dalam panggilan API mendatang, Anda dapat memberi nama pesan
dengan menyetel kolom messageId
dalam permintaan messages.create()
Anda.
Memberi nama pesan memungkinkan Anda menentukan pesan tanpa perlu menyimpan
ID yang ditetapkan sistem dari nama resource pesan (diwakili dalam
name
).
Misalnya, untuk mengambil pesan menggunakan metode get()
, Anda menggunakan
nama resource untuk menetapkan pesan yang akan diambil. Nama resource-nya adalah
diformat sebagai spaces/{space}/messages/{message}
, dengan {message}
mewakili
ID yang ditetapkan sistem atau nama kustom yang Anda tetapkan saat membuat
untuk membuat pesan email baru.
Untuk memberi nama pesan, tentukan ID kustom di
messageId
saat Anda membuat pesan. Kolom messageId
menetapkan nilai untuk
clientAssignedMessageId
pada kolom resource Message
.
Anda hanya dapat memberi nama pesan saat membuat pesan. Anda tidak dapat memberi nama atau mengubah ID kustom untuk pesan yang ada. ID kustom harus memenuhi persyaratan berikut persyaratan:
- Diawali dengan
client-
. Misalnya,client-custom-name
adalah domain kustom yang valid ID, tetapicustom-name
tidak. - Berisi maksimal 63 karakter dan hanya huruf kecil, angka, dan tanda hubung.
- Unik dalam ruang. Aplikasi Chat tidak dapat menggunakan ID kustom yang sama untuk pesan yang berbeda.
Kode berikut menunjukkan contoh cara aplikasi Chat dapat mengirim pesan teks dengan ID ke ruang tertentu atas nama pengguna terautentikasi:
Node.js
Python
Java
Apps Script
Untuk menjalankan contoh ini, ganti kode berikut:
SPACE_NAME
: ID dariname
kolom tersebut. Anda bisa mendapatkan ID dengan memanggil Metodespaces.list()
atau dari URL ruang.MESSAGE-ID
: nama untuk pesan yang dimulai dengancustom-
. Harus unik dari nama pesan lainnya yang dibuat oleh Aplikasi Chat di ruang yang ditentukan.
Memecahkan masalah
Saat aplikasi Google Chat atau kartu menampilkan error, Antarmuka Chat menampilkan pesan yang bertuliskan "Terjadi masalah". atau "Tidak dapat memproses permintaan Anda". Terkadang UI Chat tidak menampilkan pesan error apa pun, tetapi aplikasi Chat atau memberikan hasil yang tidak diharapkan; misalnya, pesan kartu mungkin tidak akan muncul.
Meskipun pesan error mungkin tidak ditampilkan di UI Chat, pesan error deskriptif dan data log tersedia untuk membantu Anda memperbaiki error saat logging error untuk aplikasi Chat diaktifkan. Untuk bantuan melihat, men-debug, dan memperbaiki error, melihat Memecahkan masalah dan memperbaiki error Google Chat.
Topik terkait
- Menggunakan Card Builder untuk mendesain dan melihat pratinjau pesan kartu JSON untuk aplikasi Chat.
- Memformat pesan.
- Mendapatkan detail tentang pesan.
- Mencantumkan pesan dalam ruang.
- Memperbarui pesan.
- Menghapus pesan.
- Mengidentifikasi pengguna dalam pesan Google Chat.
- Kirim pesan ke Google Chat dengan webhook masuk.