Visualizza il codice sorgente completo dell'esempio
Questo esempio mostra come personalizzare gli indicatori nei seguenti modi: aggiungi il testo del titolo, scala l'indicatore, cambia il colore dello sfondo, cambia il colore del bordo, cambia il colore del glifo, nascondi il glifo.
TypeScript
const parser = new DOMParser(); async function initMap() { // Request needed libraries. const { Map } = await google.maps.importLibrary("maps") as google.maps.MapsLibrary; const { AdvancedMarkerElement, PinElement } = await google.maps.importLibrary("marker") as google.maps.MarkerLibrary; const map = new Map(document.getElementById('map') as HTMLElement, { center: { lat: 37.419, lng: -122.02 }, zoom: 14, mapId: '4504f8b37365c3d0', }); // Each PinElement is paired with a MarkerView to demonstrate setting each parameter. // Default marker with title text (no PinElement). const markerViewWithText = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.03 }, title: 'Title text for the marker at lat: 37.419, lng: -122.03', }); // Adjust the scale. const pinScaled = new PinElement({ scale: 1.5, }); const markerViewScaled = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.02 }, content: pinScaled.element, }); // Change the background color. const pinBackground = new PinElement({ background: '#FBBC04', }); const markerViewBackground = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.01 }, content: pinBackground.element, }); // Change the border color. const pinBorder = new PinElement({ borderColor: '#137333', }); const markerViewBorder = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.035 }, content: pinBorder.element, }); // Change the glyph color. const pinGlyph = new PinElement({ glyphColor: 'white', }); const markerViewGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.025 }, content: pinGlyph.element, }); const pinTextGlyph = new PinElement({ glyph: 'T', glyphColor: 'white', }); const markerViewGlyphText = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.015 }, content: pinTextGlyph.element, }); // Hide the glyph. const pinNoGlyph = new PinElement({ glyph: '', }); const markerViewNoGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.005 }, content: pinNoGlyph.element, }); } initMap();
JavaScript
const parser = new DOMParser(); async function initMap() { // Request needed libraries. const { Map } = await google.maps.importLibrary("maps"); const { AdvancedMarkerElement, PinElement } = await google.maps.importLibrary( "marker", ); const map = new Map(document.getElementById("map"), { center: { lat: 37.419, lng: -122.02 }, zoom: 14, mapId: "4504f8b37365c3d0", }); // Each PinElement is paired with a MarkerView to demonstrate setting each parameter. // Default marker with title text (no PinElement). const markerViewWithText = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.03 }, title: "Title text for the marker at lat: 37.419, lng: -122.03", }); // Adjust the scale. const pinScaled = new PinElement({ scale: 1.5, }); const markerViewScaled = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.02 }, content: pinScaled.element, }); // Change the background color. const pinBackground = new PinElement({ background: "#FBBC04", }); const markerViewBackground = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.01 }, content: pinBackground.element, }); // Change the border color. const pinBorder = new PinElement({ borderColor: "#137333", }); const markerViewBorder = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.035 }, content: pinBorder.element, }); // Change the glyph color. const pinGlyph = new PinElement({ glyphColor: "white", }); const markerViewGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.025 }, content: pinGlyph.element, }); const pinTextGlyph = new PinElement({ glyph: "T", glyphColor: "white", }); const markerViewGlyphText = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.015 }, content: pinTextGlyph.element, }); // Hide the glyph. const pinNoGlyph = new PinElement({ glyph: "", }); const markerViewNoGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.005 }, content: pinNoGlyph.element, }); } initMap();
CSS
/* * Always set the map height explicitly to define the size of the div element * that contains the map. */ #map { height: 100%; } /* * Optional: Makes the sample page fill the window. */ html, body { height: 100%; margin: 0; padding: 0; }
HTML
<html> <head> <title>Advanced Marker Basic Customization</title> <link rel="stylesheet" type="text/css" href="./style.css" /> <script type="module" src="./index.js"></script> </head> <body> <div id="map"></div> <!-- prettier-ignore --> <script>(g=>{var h,a,k,p="The Google Maps JavaScript API",c="google",l="importLibrary",q="__ib__",m=document,b=window;b=b[c]||(b[c]={});var d=b.maps||(b.maps={}),r=new Set,e=new URLSearchParams,u=()=>h||(h=new Promise(async(f,n)=>{await (a=m.createElement("script"));e.set("libraries",[...r]+"");for(k in g)e.set(k.replace(/[A-Z]/g,t=>"_"+t[0].toLowerCase()),g[k]);e.set("callback",c+".maps."+q);a.src=`https://maps.${c}apis.com/maps/api/js?`+e;d[q]=f;a.onerror=()=>h=n(Error(p+" could not load."));a.nonce=m.querySelector("script[nonce]")?.nonce||"";m.head.append(a)}));d[l]?console.warn(p+" only loads once. Ignoring:",g):d[l]=(f,...n)=>r.add(f)&&u().then(()=>d[l](f,...n))}) ({key: "AIzaSyB41DRUbKWJHPxaFjMAwdrzWzbVKartNGg", v: "weekly"});</script> </body> </html>
Prova Sample
Gli indicatori avanzati utilizzano due classi per definirli: la classe AdvancedMarkerElement
fornisce i parametri di base (position
,title
e map
) e la classe PinElement
contiene opzioni per ulteriori personalizzazioni.
Per aggiungere indicatori a una mappa, devi prima
caricare la libreria marker
che fornisce le classi AdvancedMarkerElement
e PinElement
.
Lo snippet seguente mostra il codice per creare un nuovo PinElement
e poi applicarlo a un indicatore.
// Create a pin element.
const pin = new PinElement({
scale: 1.5,
});
// Create a marker and apply the element.
const marker = new AdvancedMarkerElement({
map,
position: { lat: 37.419, lng: -122.02 },
content: pin.element,
});
Nelle mappe create utilizzando HTML, i parametri di base di un indicatore vengono dichiarati utilizzando
l'elemento HTML gmp-advanced-marker
; qualsiasi personalizzazione che utilizza la
classe PinElement
deve essere applicata tramite programmazione. A questo scopo, il codice deve recuperare gli elementi gmp-advanced-marker
dalla pagina HTML. Lo snippet seguente mostra il codice per eseguire una query su una raccolta di elementi gmp-advanced-marker
, quindi esegui l'iterazione dei risultati per applicare la personalizzazione dichiarata nel PinElement
.
// Return an array of markers.
const advancedMarkers = [...document.querySelectorAll('gmp-advanced-marker')];
// Loop through the markers
for (let i = 0; i < advancedMarkers.length; i++) {
const pin = new PinElement({
scale: 2.0,
});
marker.appendChild(pin.element);
}
Questa pagina mostra come personalizzare gli indicatori nei seguenti modi:
- Aggiungere il testo del titolo
- Scalare l'indicatore
- Cambiare il colore dello sfondo
- Modificare il colore del bordo
- Modificare il colore del glifo
- Utilizzare il testo in un glifo
- Nascondere il glifo
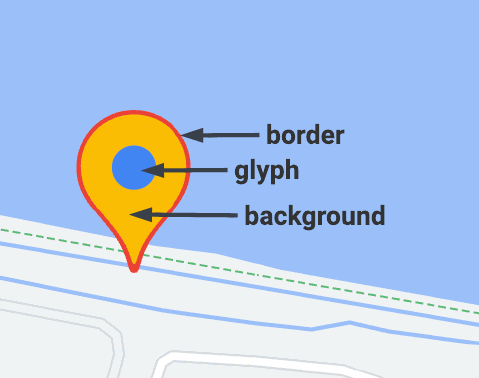
Aggiungi il testo del titolo
Il testo del titolo viene visualizzato quando il cursore passa sopra un indicatore. Il testo del titolo è leggibile dagli screen reader.
Per aggiungere il testo del titolo in modo programmatico, utilizza l'opzione AdvancedMarkerElement.title
:
// Default marker with title text (no PinElement). const markerViewWithText = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.03 }, title: "Title text for the marker at lat: 37.419, lng: -122.03", });
Per aggiungere il testo del titolo a un indicatore creato utilizzando HTML, utilizza l'attributo title
:
<gmp-map center="43.4142989,-124.2301242" zoom="4" map-id="DEMO_MAP_ID" style="height: 400px" > <gmp-advanced-marker position="37.4220656,-122.0840897" title="Mountain View, CA" ></gmp-advanced-marker> <gmp-advanced-marker position="47.648994,-122.3503845" title="Seattle, WA" ></gmp-advanced-marker> </gmp-map>
Modificare la scala dell'indicatore
Per ridimensionare un indicatore, utilizza l'opzione scale
.
TypeScript
// Adjust the scale. const pinScaled = new PinElement({ scale: 1.5, }); const markerViewScaled = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.02 }, content: pinScaled.element, });
JavaScript
// Adjust the scale. const pinScaled = new PinElement({ scale: 1.5, }); const markerViewScaled = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.02 }, content: pinScaled.element, });
Modificare il colore dello sfondo
Utilizza l'opzione PinElement.background
per modificare il colore di sfondo di un indicatore:
TypeScript
// Change the background color. const pinBackground = new PinElement({ background: '#FBBC04', }); const markerViewBackground = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.01 }, content: pinBackground.element, });
JavaScript
// Change the background color. const pinBackground = new PinElement({ background: "#FBBC04", }); const markerViewBackground = new AdvancedMarkerElement({ map, position: { lat: 37.419, lng: -122.01 }, content: pinBackground.element, });
Modificare il colore del bordo
Utilizza l'opzione PinElement.borderColor
per modificare il colore del bordo di un indicatore:
TypeScript
// Change the border color. const pinBorder = new PinElement({ borderColor: '#137333', }); const markerViewBorder = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.035 }, content: pinBorder.element, });
JavaScript
// Change the border color. const pinBorder = new PinElement({ borderColor: "#137333", }); const markerViewBorder = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.035 }, content: pinBorder.element, });
Modificare il colore del glifo
Utilizza l'opzione PinElement.glyphColor
per modificare il colore del glifo di un indicatore:
TypeScript
// Change the glyph color. const pinGlyph = new PinElement({ glyphColor: 'white', }); const markerViewGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.025 }, content: pinGlyph.element, });
JavaScript
// Change the glyph color. const pinGlyph = new PinElement({ glyphColor: "white", }); const markerViewGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.025 }, content: pinGlyph.element, });
Aggiungere testo a un glifo
Utilizza l'opzione PinElement.glyph
per sostituire il glifo predefinito con un carattere di testo. Il glifo di testo del PinElement
si adatta alle dimensioni del PinElement
e il suo colore predefinito corrisponde al glyphColor
predefinito del PinElement
.
TypeScript
const pinTextGlyph = new PinElement({ glyph: 'T', glyphColor: 'white', }); const markerViewGlyphText = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.015 }, content: pinTextGlyph.element, });
JavaScript
const pinTextGlyph = new PinElement({ glyph: "T", glyphColor: "white", }); const markerViewGlyphText = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.015 }, content: pinTextGlyph.element, });
Nascondere il glifo
Imposta l'opzione PinElement.glyph
su una stringa vuota per nascondere il glifo di un indicatore:
TypeScript
// Hide the glyph. const pinNoGlyph = new PinElement({ glyph: '', }); const markerViewNoGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.005 }, content: pinNoGlyph.element, });
JavaScript
// Hide the glyph. const pinNoGlyph = new PinElement({ glyph: "", }); const markerViewNoGlyph = new AdvancedMarkerElement({ map, position: { lat: 37.415, lng: -122.005 }, content: pinNoGlyph.element, });
In alternativa, imposta PinElement.glyphColor
sullo stesso valore di PinElement.background
.
In questo modo, il glifo viene nascosto visivamente.