소개
마커는 지도에서 위치를 나타냅니다. 기본적으로 마커는 표준 이미지를 사용합니다. 마커는 맞춤 이미지를 표시할 수 있으며 이 경우 일반적으로 마커를 '아이콘'이라고 합니다. 마커와 아이콘은 Marker
유형의 객체입니다. 마커의 생성자 내에서 또는 마커에서 setIcon()
을 호출하여 맞춤 아이콘을 설정할 수 있습니다. 마커 이미지 맞춤설정에 대해 자세히 알아보세요.
넓은 관점에서 마커는 오버레이의 한 유형입니다. 다른 오버레이 유형에 대한 자세한 내용은 지도에서 그리기를 참고하세요.
마커는 상호작용이 가능합니다. 예를 들어 마커는 기본적으로 'click'
이벤트를 수신하므로 이벤트 리스너를 추가하여 맞춤 정보가 포함된 정보 창을 표시할 수 있습니다. 마커의 draggable
속성을 true
로 설정하여 사용자가 지도에서 마커를 이동하도록 허용할 수 있습니다. 드래그 가능한 마커에 대한 자세한 내용은 아래를 참고하세요.
마커 추가
google.maps.Marker
생성자는 단일 Marker options
객체 리터럴을 가져오고 마커의 초기 속성을 지정합니다.
다음은 마커를 생성할 때 특히 중요하고 일반적으로 설정되는 필드입니다.
-
position
(필수)은 마커의 초기 위치를 나타내는LatLng
를 지정합니다.LatLng
를 가져오는 한 가지 방법은 지오코딩 서비스를 사용하는 것입니다. -
map
(선택사항)은 마커를 배치할Map
을 지정합니다. 마커를 생성할 때 지도를 지정하지 않으면 마커가 생성되지만 지도에 연결되거나 표시되지 않습니다. 마커의setMap()
메서드를 호출하여 나중에 마커를 추가할 수도 있습니다.
다음 예에서는 오스트레일리아 중앙의 울루루 지도에 간단한 마커를 추가합니다.
TypeScript
function initMap(): void { const myLatLng = { lat: -25.363, lng: 131.044 }; const map = new google.maps.Map( document.getElementById("map") as HTMLElement, { zoom: 4, center: myLatLng, } ); new google.maps.Marker({ position: myLatLng, map, title: "Hello World!", }); } declare global { interface Window { initMap: () => void; } } window.initMap = initMap;
JavaScript
function initMap() { const myLatLng = { lat: -25.363, lng: 131.044 }; const map = new google.maps.Map(document.getElementById("map"), { zoom: 4, center: myLatLng, }); new google.maps.Marker({ position: myLatLng, map, title: "Hello World!", }); } window.initMap = initMap;
샘플 사용해 보기
위 예에서는 마커가 마커 옵션의 map
속성을 사용하여 마커를 생성할 때 지도에 배치됩니다.
또는 아래 예에서처럼 마커의 setMap()
메서드를 사용하여 지도에 직접 마커를 추가할 수도 있습니다.
var myLatlng = new google.maps.LatLng(-25.363882,131.044922); var mapOptions = { zoom: 4, center: myLatlng } var map = new google.maps.Map(document.getElementById("map"), mapOptions); var marker = new google.maps.Marker({ position: myLatlng, title:"Hello World!" }); // To add the marker to the map, call setMap(); marker.setMap(map);
마커의 title
은 도움말로 표시됩니다.
마커의 생성자에 Marker options
를 전달하지 않으려면 생성자의 마지막 인수에서 빈 객체({}
)를 대신 전달하세요.
마커 제거
지도에서 마커를 제거하려면 null
을 인수로 전달하는 setMap()
메서드를 호출하세요.
marker.setMap(null);
위의 메서드는 마커를 삭제하지 않습니다. 지도에서 마커를 제거할 뿐입니다. 마커를 삭제하려면 지도에서 제거한 다음 마커 자체를 null
로 설정해야 합니다.
마커 집합을 관리하려면 마커를 보유하는 배열을 만들어야 합니다. 이 배열을 사용하면 마커를 제거해야 할 때 배열의 각 마커에서 setMap()
을 차례로 호출할 수 있습니다. 지도에서 마커를 제거한 후 배열의 length
를 0
으로 설정하여 마커를 삭제할 수 있습니다. 이렇게 하면 마커에 대한 모든 참조가 제거됩니다.
마커 이미지 맞춤설정
기본 Google 지도 압정 아이콘 대신 표시할 이미지 파일 또는 벡터 기반 아이콘을 지정하여 마커의 시각적 모양을 맞춤설정할 수 있습니다. 마커 라벨이 있는 텍스트를 추가하고, 복잡한 아이콘을 사용하여 클릭 가능한 영역을 정의하고 마커의 스택 순서를 설정할 수 있습니다.
이미지 아이콘이 있는 마커
가장 기본적인 경우 아이콘은 기본 Google 지도 압정 아이콘 대신 사용할 이미지를 나타낼 수 있습니다. 이러한 아이콘을 지정하려면 마커의 icon
속성을 이미지의 URL로 설정하세요. Maps JavaScript API는 아이콘의 크기를 자동으로 지정합니다.
TypeScript
// This example adds a marker to indicate the position of Bondi Beach in Sydney, // Australia. function initMap(): void { const map = new google.maps.Map( document.getElementById("map") as HTMLElement, { zoom: 4, center: { lat: -33, lng: 151 }, } ); const image = "https://developers.google.com/maps/documentation/javascript/examples/full/images/beachflag.png"; const beachMarker = new google.maps.Marker({ position: { lat: -33.89, lng: 151.274 }, map, icon: image, }); } declare global { interface Window { initMap: () => void; } } window.initMap = initMap;
JavaScript
// This example adds a marker to indicate the position of Bondi Beach in Sydney, // Australia. function initMap() { const map = new google.maps.Map(document.getElementById("map"), { zoom: 4, center: { lat: -33, lng: 151 }, }); const image = "https://developers.google.com/maps/documentation/javascript/examples/full/images/beachflag.png"; const beachMarker = new google.maps.Marker({ position: { lat: -33.89, lng: 151.274 }, map, icon: image, }); } window.initMap = initMap;
샘플 사용해 보기
벡터 기반 아이콘이 있는 마커
맞춤 SVG 벡터 경로를 사용하여 마커의 시각적 모양을 정의할 수 있습니다. 이렇게 하려면 Symbol
객체 리터럴을 원하는 경로와 함께 마커의 icon
속성에 전달하세요. SVG 경로 표기법을 사용하여 맞춤 경로를 정의하거나 google.maps.SymbolPath의 사전 정의된 경로 중 하나를 사용할 수 있습니다. 확대/축소 수준이 변경될 때 마커가 올바르게 렌더링되려면 anchor
속성이 필요합니다. 마커 및 다중선에 대해 기호를 사용하여 벡터 기반 아이콘을 만드는 방법에 관해 자세히 알아보세요.
TypeScript
// This example uses SVG path notation to add a vector-based symbol // as the icon for a marker. The resulting icon is a marker-shaped // symbol with a blue fill and no border. function initMap(): void { const center = new google.maps.LatLng(-33.712451, 150.311823); const map = new google.maps.Map( document.getElementById("map") as HTMLElement, { zoom: 9, center: center, } ); const svgMarker = { path: "M-1.547 12l6.563-6.609-1.406-1.406-5.156 5.203-2.063-2.109-1.406 1.406zM0 0q2.906 0 4.945 2.039t2.039 4.945q0 1.453-0.727 3.328t-1.758 3.516-2.039 3.070-1.711 2.273l-0.75 0.797q-0.281-0.328-0.75-0.867t-1.688-2.156-2.133-3.141-1.664-3.445-0.75-3.375q0-2.906 2.039-4.945t4.945-2.039z", fillColor: "blue", fillOpacity: 0.6, strokeWeight: 0, rotation: 0, scale: 2, anchor: new google.maps.Point(0, 20), }; new google.maps.Marker({ position: map.getCenter(), icon: svgMarker, map: map, }); } declare global { interface Window { initMap: () => void; } } window.initMap = initMap;
JavaScript
// This example uses SVG path notation to add a vector-based symbol // as the icon for a marker. The resulting icon is a marker-shaped // symbol with a blue fill and no border. function initMap() { const center = new google.maps.LatLng(-33.712451, 150.311823); const map = new google.maps.Map(document.getElementById("map"), { zoom: 9, center: center, }); const svgMarker = { path: "M-1.547 12l6.563-6.609-1.406-1.406-5.156 5.203-2.063-2.109-1.406 1.406zM0 0q2.906 0 4.945 2.039t2.039 4.945q0 1.453-0.727 3.328t-1.758 3.516-2.039 3.070-1.711 2.273l-0.75 0.797q-0.281-0.328-0.75-0.867t-1.688-2.156-2.133-3.141-1.664-3.445-0.75-3.375q0-2.906 2.039-4.945t4.945-2.039z", fillColor: "blue", fillOpacity: 0.6, strokeWeight: 0, rotation: 0, scale: 2, anchor: new google.maps.Point(0, 20), }; new google.maps.Marker({ position: map.getCenter(), icon: svgMarker, map: map, }); } window.initMap = initMap;
샘플 사용해 보기
마커 라벨
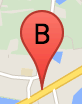
마커 라벨은 마커 안에 표시되는 문자 또는 숫자입니다. 이 섹션의 마커 이미지는 문자 'B'가 있는 마커 라벨을 표시합니다. 마커 라벨을 문자열이나 문자열 및 기타 라벨 속성이 포함된 MarkerLabel
객체로 지정할 수 있습니다.
마커를 만들 때 MarkerOptions
객체에 label
속성을 지정할 수 있습니다. 또는 마커 객체의 setLabel()
을 호출하여 기존 마커에 라벨을 설정할 수 있습니다.
다음 예에서는 사용자가 지도를 클릭할 때 라벨이 지정된 마커를 표시합니다.
TypeScript
// In the following example, markers appear when the user clicks on the map. // Each marker is labeled with a single alphabetical character. const labels = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"; let labelIndex = 0; function initMap(): void { const bangalore = { lat: 12.97, lng: 77.59 }; const map = new google.maps.Map( document.getElementById("map") as HTMLElement, { zoom: 12, center: bangalore, } ); // This event listener calls addMarker() when the map is clicked. google.maps.event.addListener(map, "click", (event) => { addMarker(event.latLng, map); }); // Add a marker at the center of the map. addMarker(bangalore, map); } // Adds a marker to the map. function addMarker(location: google.maps.LatLngLiteral, map: google.maps.Map) { // Add the marker at the clicked location, and add the next-available label // from the array of alphabetical characters. new google.maps.Marker({ position: location, label: labels[labelIndex++ % labels.length], map: map, }); } declare global { interface Window { initMap: () => void; } } window.initMap = initMap;
JavaScript
// In the following example, markers appear when the user clicks on the map. // Each marker is labeled with a single alphabetical character. const labels = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"; let labelIndex = 0; function initMap() { const bangalore = { lat: 12.97, lng: 77.59 }; const map = new google.maps.Map(document.getElementById("map"), { zoom: 12, center: bangalore, }); // This event listener calls addMarker() when the map is clicked. google.maps.event.addListener(map, "click", (event) => { addMarker(event.latLng, map); }); // Add a marker at the center of the map. addMarker(bangalore, map); } // Adds a marker to the map. function addMarker(location, map) { // Add the marker at the clicked location, and add the next-available label // from the array of alphabetical characters. new google.maps.Marker({ position: location, label: labels[labelIndex++ % labels.length], map: map, }); } window.initMap = initMap;
샘플 사용해 보기
복잡한 아이콘
복잡한 도형을 지정하여 클릭 가능한 영역을 표시하고 다른 오버레이에 비례하여 아이콘이 표시되는 방식('스택 순서')을 지정할 수 있습니다. 이 방식으로 지정된 아이콘은 icon
속성을 Icon
유형의 객체로 설정해야 합니다.
Icon
객체는 이미지를 정의합니다. 이 객체는 또한 아이콘의 size
, 아이콘의 origin
(예를 들어 원하는 이미지가 스프라이트에 있는 더 큰 이미지의 일부인 경우), (원점에 기반을 둔) 아이콘의 핫스팟을 배치해야 하는 anchor
를 정의합니다.
맞춤 마커와 함께 라벨을 사용하면 Icon
객체의 labelOrigin
속성을 사용하여 라벨을 배치할 수 있습니다.
TypeScript
// The following example creates complex markers to indicate beaches near // Sydney, NSW, Australia. Note that the anchor is set to (0,32) to correspond // to the base of the flagpole. function initMap(): void { const map = new google.maps.Map( document.getElementById("map") as HTMLElement, { zoom: 10, center: { lat: -33.9, lng: 151.2 }, } ); setMarkers(map); } // Data for the markers consisting of a name, a LatLng and a zIndex for the // order in which these markers should display on top of each other. const beaches: [string, number, number, number][] = [ ["Bondi Beach", -33.890542, 151.274856, 4], ["Coogee Beach", -33.923036, 151.259052, 5], ["Cronulla Beach", -34.028249, 151.157507, 3], ["Manly Beach", -33.80010128657071, 151.28747820854187, 2], ["Maroubra Beach", -33.950198, 151.259302, 1], ]; function setMarkers(map: google.maps.Map) { // Adds markers to the map. // Marker sizes are expressed as a Size of X,Y where the origin of the image // (0,0) is located in the top left of the image. // Origins, anchor positions and coordinates of the marker increase in the X // direction to the right and in the Y direction down. const image = { url: "https://developers.google.com/maps/documentation/javascript/examples/full/images/beachflag.png", // This marker is 20 pixels wide by 32 pixels high. size: new google.maps.Size(20, 32), // The origin for this image is (0, 0). origin: new google.maps.Point(0, 0), // The anchor for this image is the base of the flagpole at (0, 32). anchor: new google.maps.Point(0, 32), }; // Shapes define the clickable region of the icon. The type defines an HTML // <area> element 'poly' which traces out a polygon as a series of X,Y points. // The final coordinate closes the poly by connecting to the first coordinate. const shape = { coords: [1, 1, 1, 20, 18, 20, 18, 1], type: "poly", }; for (let i = 0; i < beaches.length; i++) { const beach = beaches[i]; new google.maps.Marker({ position: { lat: beach[1], lng: beach[2] }, map, icon: image, shape: shape, title: beach[0], zIndex: beach[3], }); } } declare global { interface Window { initMap: () => void; } } window.initMap = initMap;
JavaScript
// The following example creates complex markers to indicate beaches near // Sydney, NSW, Australia. Note that the anchor is set to (0,32) to correspond // to the base of the flagpole. function initMap() { const map = new google.maps.Map(document.getElementById("map"), { zoom: 10, center: { lat: -33.9, lng: 151.2 }, }); setMarkers(map); } // Data for the markers consisting of a name, a LatLng and a zIndex for the // order in which these markers should display on top of each other. const beaches = [ ["Bondi Beach", -33.890542, 151.274856, 4], ["Coogee Beach", -33.923036, 151.259052, 5], ["Cronulla Beach", -34.028249, 151.157507, 3], ["Manly Beach", -33.80010128657071, 151.28747820854187, 2], ["Maroubra Beach", -33.950198, 151.259302, 1], ]; function setMarkers(map) { // Adds markers to the map. // Marker sizes are expressed as a Size of X,Y where the origin of the image // (0,0) is located in the top left of the image. // Origins, anchor positions and coordinates of the marker increase in the X // direction to the right and in the Y direction down. const image = { url: "https://developers.google.com/maps/documentation/javascript/examples/full/images/beachflag.png", // This marker is 20 pixels wide by 32 pixels high. size: new google.maps.Size(20, 32), // The origin for this image is (0, 0). origin: new google.maps.Point(0, 0), // The anchor for this image is the base of the flagpole at (0, 32). anchor: new google.maps.Point(0, 32), }; // Shapes define the clickable region of the icon. The type defines an HTML // <area> element 'poly' which traces out a polygon as a series of X,Y points. // The final coordinate closes the poly by connecting to the first coordinate. const shape = { coords: [1, 1, 1, 20, 18, 20, 18, 1], type: "poly", }; for (let i = 0; i < beaches.length; i++) { const beach = beaches[i]; new google.maps.Marker({ position: { lat: beach[1], lng: beach[2] }, map, icon: image, shape: shape, title: beach[0], zIndex: beach[3], }); } } window.initMap = initMap;
샘플 사용해 보기
MarkerImage
객체를 Icon
유형으로 변환
Maps JavaScript API 버전 3.10까지는 복잡한 아이콘이 MarkerImage
객체로 정의되었습니다. Icon
객체 리터럴은 버전 3.10에 추가되었으며 버전 3.11부터 MarkerImage
를 대체합니다.
Icon
객체 리터럴은 MarkerImage
와 동일한 매개변수를 지원하며, 이 객체를 사용하면 생성자를 삭제하고 {}
의 이전 매개변수를 래핑하고 각 매개변수의 이름을 추가하여 MarkerImage
를 Icon
으로 쉽게 변환할 수 있습니다. 예를 들면 다음과 같습니다.
var image = new google.maps.MarkerImage( place.icon, new google.maps.Size(71, 71), new google.maps.Point(0, 0), new google.maps.Point(17, 34), new google.maps.Size(25, 25));
위 내용이 다음과 같이 변환됩니다.
var image = { url: place.icon, size: new google.maps.Size(71, 71), origin: new google.maps.Point(0, 0), anchor: new google.maps.Point(17, 34), scaledSize: new google.maps.Size(25, 25) };
마커 최적화
최적화하면 다수의 마커를 단일 정적 요소로 렌더링하므로 성능이 향상됩니다. 이는 많은 수의 마커가 필요한 경우에 유용합니다. 기본적으로 Maps JavaScript API가 마커를 최적화할지를 결정합니다. 다수의 마커가 있는 경우 Maps JavaScript API에서는 최적화를 사용하여 마커를 렌더링하려고 시도합니다. 모든 마커를 최적화할 수는 없으며, 경우에 따라 Maps JavaScript API에서 최적화 없이 마커를 렌더링해야 할 수도 있습니다. 애니메이션 GIF나 PNG를 대상으로 하거나, 각 마커를 별도의 DOM 요소로 렌더링해야 할 때에는 최적화된 렌더링을 사용 중지합니다. 다음 예는 최적화된 마커를 만드는 방법을 보여줍니다.
var marker = new google.maps.Marker({ position: myLatlng, title:"Hello World!", optimized: true });
마커에 접근성 기능 추가
클릭 리스너 이벤트를 추가하고 optimized
를 false
로 설정하여 마커에 접근성 기능을 추가할 수 있습니다. 클릭 리스너로 인해 마커에는 키보드 탐색, 스크린 리더 등을 사용하여 액세스할 수 있는 버튼 시맨틱이 생성됩니다. title
옵션을 사용하여 마커에 접근성 텍스트를 표시하세요.
다음 예에서 Tab 키를 누르면 첫 번째 마커로 포커스가 이동합니다. 그런 다음 화살표 키를 사용하여 마커 사이를 이동할 수 있습니다. 나머지 지도 컨트롤로 계속 이동하려면 Tab을 다시 누르세요. 마커에 정보 창이 있는 경우 마커를 클릭하거나 마커가 선택되었을 때 Enter 키 또는 스페이스바를 눌러 정보 창을 열 수 있습니다. 정보 창이 닫히면 포커스가 연결된 마커로 돌아갑니다.
TypeScript
// The following example creates five accessible and // focusable markers. function initMap(): void { const map = new google.maps.Map( document.getElementById("map") as HTMLElement, { zoom: 12, center: { lat: 34.84555, lng: -111.8035 }, } ); // Set LatLng and title text for the markers. The first marker (Boynton Pass) // receives the initial focus when tab is pressed. Use arrow keys to // move between markers; press tab again to cycle through the map controls. const tourStops: [google.maps.LatLngLiteral, string][] = [ [{ lat: 34.8791806, lng: -111.8265049 }, "Boynton Pass"], [{ lat: 34.8559195, lng: -111.7988186 }, "Airport Mesa"], [{ lat: 34.832149, lng: -111.7695277 }, "Chapel of the Holy Cross"], [{ lat: 34.823736, lng: -111.8001857 }, "Red Rock Crossing"], [{ lat: 34.800326, lng: -111.7665047 }, "Bell Rock"], ]; // Create an info window to share between markers. const infoWindow = new google.maps.InfoWindow(); // Create the markers. tourStops.forEach(([position, title], i) => { const marker = new google.maps.Marker({ position, map, title: `${i + 1}. ${title}`, label: `${i + 1}`, optimized: false, }); // Add a click listener for each marker, and set up the info window. marker.addListener("click", () => { infoWindow.close(); infoWindow.setContent(marker.getTitle()); infoWindow.open(marker.getMap(), marker); }); }); } declare global { interface Window { initMap: () => void; } } window.initMap = initMap;
JavaScript
// The following example creates five accessible and // focusable markers. function initMap() { const map = new google.maps.Map(document.getElementById("map"), { zoom: 12, center: { lat: 34.84555, lng: -111.8035 }, }); // Set LatLng and title text for the markers. The first marker (Boynton Pass) // receives the initial focus when tab is pressed. Use arrow keys to // move between markers; press tab again to cycle through the map controls. const tourStops = [ [{ lat: 34.8791806, lng: -111.8265049 }, "Boynton Pass"], [{ lat: 34.8559195, lng: -111.7988186 }, "Airport Mesa"], [{ lat: 34.832149, lng: -111.7695277 }, "Chapel of the Holy Cross"], [{ lat: 34.823736, lng: -111.8001857 }, "Red Rock Crossing"], [{ lat: 34.800326, lng: -111.7665047 }, "Bell Rock"], ]; // Create an info window to share between markers. const infoWindow = new google.maps.InfoWindow(); // Create the markers. tourStops.forEach(([position, title], i) => { const marker = new google.maps.Marker({ position, map, title: `${i + 1}. ${title}`, label: `${i + 1}`, optimized: false, }); // Add a click listener for each marker, and set up the info window. marker.addListener("click", () => { infoWindow.close(); infoWindow.setContent(marker.getTitle()); infoWindow.open(marker.getMap(), marker); }); }); } window.initMap = initMap;
샘플 사용해 보기
마커에 애니메이션 적용
다양한 상황에서 동적인 움직임을 보이도록 마커에 애니메이션을 적용할 수 있습니다. 마커에 애니메이션이 적용되는 방식을 지정하려면 마커의 animation
속성(google.maps.Animation
유형)을 사용하세요. 다음과 같은 Animation
값이 지원됩니다.
-
DROP
은 마커를 처음 지도에 배치할 때 지도 상단에서 최종 위치로 떨어져야 함을 나타냅니다. 마커가 멈추면 애니메이션이 중지되고animation
이null
로 돌아갑니다. 이 유형의 애니메이션은 일반적으로Marker
를 생성하는 동안 지정됩니다. -
BOUNCE
는 마커가 제자리에서 튀어야 함을 나타냅니다. 튀는 마커는animation
속성이 명시적으로null
이 될 때까지 계속 튑니다.
기존 마커의 경우 Marker
객체에서 setAnimation()
을 호출하여 애니메이션을 시작할 수 있습니다.
TypeScript
// The following example creates a marker in Stockholm, Sweden using a DROP // animation. Clicking on the marker will toggle the animation between a BOUNCE // animation and no animation. let marker: google.maps.Marker; function initMap(): void { const map = new google.maps.Map( document.getElementById("map") as HTMLElement, { zoom: 13, center: { lat: 59.325, lng: 18.07 }, } ); marker = new google.maps.Marker({ map, draggable: true, animation: google.maps.Animation.DROP, position: { lat: 59.327, lng: 18.067 }, }); marker.addListener("click", toggleBounce); } function toggleBounce() { if (marker.getAnimation() !== null) { marker.setAnimation(null); } else { marker.setAnimation(google.maps.Animation.BOUNCE); } } declare global { interface Window { initMap: () => void; } } window.initMap = initMap;
JavaScript
// The following example creates a marker in Stockholm, Sweden using a DROP // animation. Clicking on the marker will toggle the animation between a BOUNCE // animation and no animation. let marker; function initMap() { const map = new google.maps.Map(document.getElementById("map"), { zoom: 13, center: { lat: 59.325, lng: 18.07 }, }); marker = new google.maps.Marker({ map, draggable: true, animation: google.maps.Animation.DROP, position: { lat: 59.327, lng: 18.067 }, }); marker.addListener("click", toggleBounce); } function toggleBounce() { if (marker.getAnimation() !== null) { marker.setAnimation(null); } else { marker.setAnimation(google.maps.Animation.BOUNCE); } } window.initMap = initMap;
샘플 사용해 보기
마커가 여러 개인 경우 모든 마커를 동시에 지도에 배치하고 싶지 않을 수도 있습니다. setTimeout()
을 활용하면 아래에 표시된 것과 같은 패턴을 사용하여 마커의 애니메이션 사이에 간격을 둘 수 있습니다.
function drop() { for (var i =0; i < markerArray.length; i++) { setTimeout(function() { addMarkerMethod(); }, i * 200); } }
마커를 드래그할 수 있도록 설정
사용자가 마커를 지도의 다른 위치로 드래그할 수 있도록 하려면 도형 옵션에서 draggable
을 true
로 설정하세요.
var myLatlng = new google.maps.LatLng(-25.363882,131.044922); var mapOptions = { zoom: 4, center: myLatlng } var map = new google.maps.Map(document.getElementById("map"), mapOptions); // Place a draggable marker on the map var marker = new google.maps.Marker({ position: myLatlng, map: map, draggable:true, title:"Drag me!" });
마커 추가 맞춤설정
완전히 맞춤설정된 마커는 맞춤설정된 팝업 예를 참고하세요.
마커 클래스의 추가 확장, 마커 클러스터링 및 관리, 오버레이 맞춤설정에 대해서는 오픈소스 라이브러리를 참고하세요.